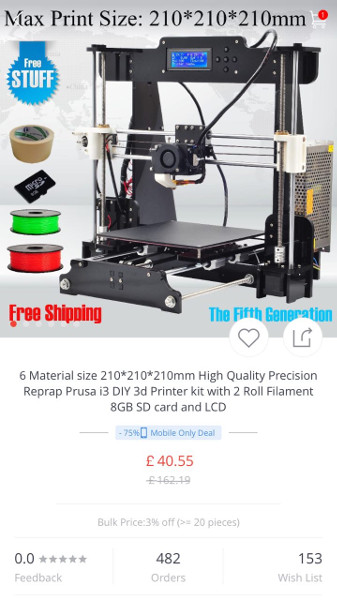
When i saw this, I thought "£40 for a 3d printer? Including UPS
shipping? This is too good to be true!"
Though thinking about it a bit more, I couldn't think of any way they
could get away with the money. Aliexpress offers full buyer protection
if you buy through alipay, and money is held in escrow. So I bought a
couple to see what the dealio is. If it does arrive and its shit, just
the motors would cost more then £40 to buy, and I can definitely reuse
them. I figured going into this paranoid, I might just about be able to
figure it out before they run away with my money.
This morning, I got shipping confirmation, with a china post shipping
code. "Wow, maybe its not a scam?" I thought. Then I received this
message:
hi. friend. Because you choose courier suspended. We send you the
goods by China Post Air Parcel. Give you cause delays receive the
package, so we give you $15 in compensation. You may submit
disputes. A partial refund of $15 agreement. We give you a refund in
a timely manner. Thank you
Ok, yeah, thats a bit odd. This is the penny dropping.
The way AliExpress works, you can open a dispute on any order for either
the full, or part of the purchase price. If the seller agrees, the
refund is paid out to you right away. But if I open a dispute for the
shipping, maybe I lose the ability to open a dispute for the remainder
if the item doesn't arrive? The FAQs were unclear, so I set off to
AliExpress customer support:
If I open a dispute for a partial refund (for the shipping), can I then open another dispute later if the item does not arrive
No, if the dispute has been agreed the seller with partial refund, the
remaining payment will be release to the seller automatically
With this, I highly suggest to please ask a full refund instead.
So there it is. Beautiful in its simplicity. You ask for a dispute for
the shipping charges, get your £8 shipping back, and lose the ability to
claim back your £32 for the rest the item when it doesnt arrive. Since
I have 40 days of purchase protection remaining, I'm going to hold off
claiming a full refund for a couple of weeks, and I'll update here if I
turn out to be wrong and something does arrive!
Update!
Another message is being sent to those of us who have ignored the first
message, or replied that the refund is not necessary:
Friends. Goods are in transit. Do not worry. We give you a refund of
compensation today. Tomorrow no longer compensate, please submit the
dispute in a timely manner, thank you
Ahh, a time limit! Best get the refund quick, otherwise they wont offer
it anymore! I've received this second message, as have others. I'm
guessing it wont be long until aliexpress close their account, so
they've gotta try and get as much cash out asap.
Update!
Just got an email from aliexpress:
Your order --REDACTED-- has been frozen due to suspicious
seller activity.
We have suspended this seller’s account because we detected unsafe
trading activities. All of your pending orders with this seller have
been frozen for your security.
We have asked this supplier to provide supporting evidence, such as
shipping documents and qualification certificates. If the supplier is
unable to provide the evidence or if the evidence is insufficient, we
will close the order and process the refund for you within 5 business
days (In case of certain circumstance, the processing period may be
extended).
We apologize for this inconvenience. At AliExpress we are committed to
ensuring you enjoy a safe shopping experience. We will continue
striving to improve. Your understanding and cooperation are highly
appreciated, if you have any question or suggestion, please click here
Your understanding and cooperation are highly appreciated.
Sincerely,
AliExpress Trade Security Department
I'm fairly unsurprised by this, hopefully the refund will be forthcoming
fairly quickly.
Final Update
Your Order No:-REDACTED- has been closed because the supplier did not
provide necessary evidence. The payment will be refunded to you within
7-10 working days.
AliExpress strives to continuously improve our trading environment.
Thank you for your understanding and continued support.
Sincerely,
AliExpress Trade Security Department
And its over. This experience has massively reaffirmed my confidence in
aliexpress' customer services and payment systems. Other sales websites
should take notice!